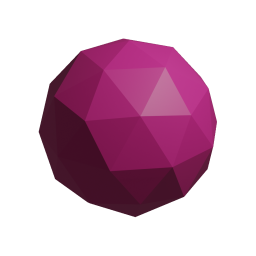
AngouriMath
Compilation
We will consider ways to improve the performance of computations via compiling functions.
Compilation into a native delegate
Here we will consider a bunch of methods, which all are overloads of one:Entity expr = "sin(x)";
Func mySin = expr.Compile("x");
Console.WriteLine(mySin(4.2f));
Output:
-0.87157565
The variable Entity expr = "sqrt(sin(x) + 3)";
var f = expr.Compile("x");
Console.WriteLine(f(4.2f));
Output:
1.458912
The number Entity expr = "sqrt(sin(x) + 3)";
var f = expr.Compile("x");
Console.WriteLine(f(4));
Output:
1
You can also pass multiple parameters, as well as multiple parameters of different types. Example:
Entity expr = "sin(x) + y";
var f = expr.Compile("x", "y");
Console.WriteLine(f(4.3f, 7.8d));
Output:
6.883834
The supported types are: Entity expr = "x + y";
var f = expr.Compile("x", "y");
Console.WriteLine(f(new(4, 5), 5));
Output:
(9, 5)
You can pass up to 8 type parameters excluding the one for output.
Extensions include the same set of methods, but for string.
If needed to pass more than 8 parameters, or other types than the ones listed, or
custom rules for types, consider declaring your own CompilationProtocol
Since the way AM compiles an expression is through Linq.Expression, it requires provided rules for constants to be converted into a constant, unary/binary/n-ary nodes to be converted into calls or operators. That is why AM has class...
return typeHolder switch
{
Sumf => Expression.Add(left, right),
Minusf => Expression.Subtract(left, right),
Mulf => Expression.Multiply(left, right),
Divf => Expression.Divide(left, right),
...
Same way it is recommended to define Entity expr = "x + y";
var f = expr.Compile>(
new(), typeof(float),
new[] { (typeof(float), Var("x")), (typeof(float), Var("y")) }
);
Console.WriteLine(f(4, 5));
Here we use Compilation into FastExpression
It is highly recommend to compile a given expression the way it is described above.Entity expr = "x + y";
var f = expr.Compile("x", "y");
Console.WriteLine(f.Call(4, 5));
Output:
(9, 0)
It is not as fast and flexible as Angouri © 2019-2023 · Project's repo · Site's repo · Octicons · Transparency · 1534 pages online